Event Driven Programming Model
One of the most attractive aspects of the .Net Grid is that in addition to standard modes it also supports event-driven programming model with thread protection.
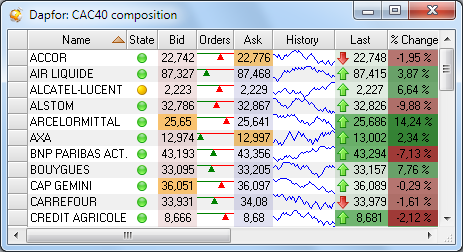
What is it and what is it for?
In .Net 1.0 a very important interface was added to the System.ComponentModel namespace – INotifyPropertyChanged:
public interface INotifyPropertyChanged
{
event PropertyChangedEventHandler PropertyChanged;
}
It is used to notify clients(typically binding clients) that a property value has changed. .Net Grid can bind to a data source for implementing such operations as automated real-time updates, sorting, filtering and data grouping. It’s important to emphasize that all notifications are synchronized by the .Net Grid with GUI thread, which in turn means that data can be changed not only in GUI thread but also in any other thread, safely reporting to the .Net Grid about value changes.
Why is it convenient?
A typical application consists of a set of classes that represent its business logic. In object-oriented programming this logic should always be present in some form. This business logic is represented by rows in the .Net Grid without regard to specific model used. When a programmer changes data object values in non-event-driven data grids, he has to find rows in the data grid and sort them, group them and verify their filtering criteria. It’s important to remember that in well-designed applications it is necessary to take care about thread safety. In event-driven model, a data grid receives notifications about data changes and performs all the above mentioned operations.
public class Product : INotifyPropertyChanged
{
private double price;
private DateTime maturity;
[DoubleFormat(Precision = 3, ShortForm = true, ShowZero = false)]
public double Price
{
get { return price; }
set
{
price = value;
if(PropertyChanged != null)
{
PropertyChanged(this, new PropertyChangedEventArgs("Price"));
}
}
}
public DateTime Maturity
{
get { return maturity; }
}
public event PropertyChangedEventHandler PropertyChanged;
}
Product product = new Product();
Row row = grid.Rows.Add(product);
row.Add(new OtherDataObject());
product.Price = 123;
Three important advantages of using event-driven model:
- From the foregoing example it’s clear that objects implement standard INotifyPropertyChanged interface which has only one public event – PropertyChanged. When business layer values are changed, you don't need to seek rows in data grid. Instead, .Net Grid will perform all necessary actions like sorting, filtering, grouping, invalidating and highlighting of the data. It means that business logic layer doesn’t depend on the presentation layer. In a perfect case, your assembly will contain no business logic dependencies with any other assemblies that contain references to System.Windows.Forms or any .Net Grid that is able to receive notifications via INotifyPropertyChanged interface.
- If you application is multi-threaded and modifies data in non-GUI thread,
the most common way to synchronize threads is to invoke
Control.Invoke()/Control.BeginInvoke() methods. In other words, you have to add quite dubious dependencies from graphic user interface and from System.Windows.Forms to your business logic layer, especially to provide means of synchronization. - Event-driven model is more efficient as combined to other models, specifically because. You don't have to perform pointless searching of rows that correspond to business data. Any profiler shall demonstrate that after data painting in GDI/GDI+ the most resource-intensive operation in your application is the recalculation of data in specific grid positions and vice-versa. In many cases CPU load is directly proportional to the number of rows in the grid.
It’s important to keep in mind, that implementation of event-driven model considerably simplifies transition to another technologies such as WPF or Silverlight, as you don’t need to change your business logic layer – the only thing you have to do is to make slight modifications in the presentation layer.
Back to .Net Grid Features