Conditional Data Binding
Starting from version 2.5.0 .Net grid provides new data binding engines in addition to already existing
- Binding to multiple sources
- Hierarchical binding based on hierarchy fields of data objects
- Binding on any hierarchy level with Row.DataSource
- Conditional binding
Conditional binding is one of the most powerful and convenient types of binding. It is an API provided by the grid that enables the programmer to specify insertion method when a data object is added to the grid.
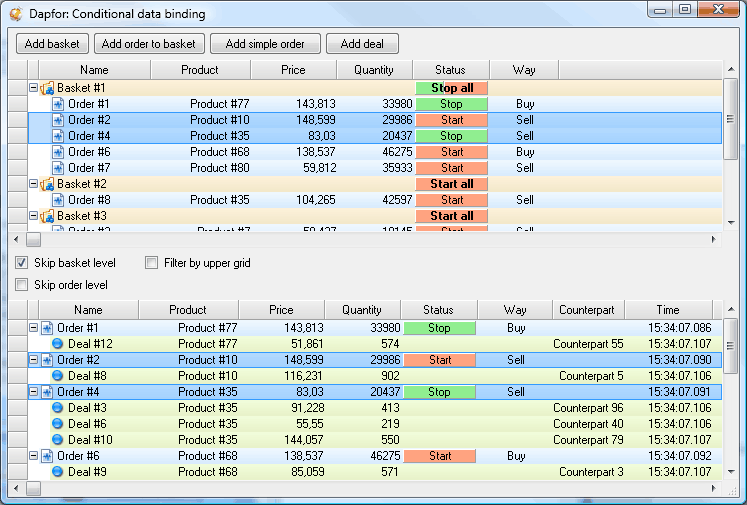
This API is implemented as an event that is called when data is added to the grid.
public class Grid
{
public event EventHandler<GridRowAddingEventArgs> RowAdding;
}
This event contains a reference to the added object, a parent row if
any and insertion method for this object. Below is the list of possible
actions upon data insertion to the grid:
- AsChild - regular method of data object insertion to parent. A programmer may replace the inserted object with any other object on his own discretion.
- AsCollection - a programmer may replace a data object with any collection that shall be added instead of the specified object. If the inserted basket contains multiple orders, the grid shall skip the basket and add collection instead.
- AsChildAndCollection - the grid adds a data object and a new collection. The data object is added to the specified parent as with AsChild, and the collection is added on the next hierarchy level to the data object that has just been added.
- Skip - the data object is not added to the grid.
It is important to note that if a collection implements
IBindingList interface, the grid subscribes to events of this collection and inserts or modifies data on corresponding hierarchy level of the grid. All binding list operations are thread safe.
public class Basket
{
private readonly BindingList<Order> _orders = new BindingList<Order>();
public IList<Order> Orders
{
get { return _orders; }
}
}
public void InitializeGrid(Grid grid, IList<Basket> baskets)
{
grid.RowAdding += OnGridRowAdding;
grid.DataSource = baskets;
}
private void OnGridRowAdding(object sender, GridRowAddingEventArgs e)
{
Basket basket = e.DataObject as Basket;
if (basket != null)
{
e.DataObject = basket.Orders;
e.InsertionType = InsertionType.AsCollection;
}
}
Back to .Net Grid Features